Dual Doppler Analysis
Contents
Dual Doppler Analysis¶
import pyart
import pydda
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import glob
## You are using the Python ARM Radar Toolkit (Py-ART), an open source
## library for working with weather radar data. Py-ART is partly
## supported by the U.S. Department of Energy as part of the Atmospheric
## Radiation Measurement (ARM) Climate Research Facility, an Office of
## Science user facility.
##
## If you use this software to prepare a publication, please cite:
##
## JJ Helmus and SM Collis, JORS 2016, doi: 10.5334/jors.119
# read in the CSU sounding for this case
import xarray as xr
ds = xr.open_dataset('../../Downloads/CSU_griddedsounding.nc')
ds.sel(launch_time='2018-11-10').launch_time.values
array(['2018-11-10T22:09:00.000000000', '2018-11-10T21:04:00.000000000',
'2018-11-10T20:01:00.000000000', '2018-11-10T20:00:00.000000000',
'2018-11-10T18:06:00.000000000', '2018-11-10T17:04:00.000000000',
'2018-11-10T16:02:00.000000000', '2018-11-10T15:00:00.000000000'],
dtype='datetime64[ns]')
sounding_ds = ds.sel(launch_time = ds.sel(launch_time='2018-11-10').launch_time.values[4]).dropna('pressure')
uwnd = sounding_ds.Ucmp.values
vwnd = sounding_ds.Vcmp.values
alt = sounding_ds.Alt.values
profile = pyart.core.HorizontalWindProfile.from_u_and_v(alt, uwnd, vwnd)
def plot_radars(radar):
display = pyart.graph.RadarDisplay(radar)
fig = plt.figure(figsize=(20, 20))
nyq = radar.instrument_parameters['nyquist_velocity']['data'][0]
# Reflectivity
ax = fig.add_subplot(221)
display.plot('DBZHC_F', 1, vmin=-32, vmax=64., cmap='pyart_HomeyerRainbow')
display.plot_range_rings([10, 20, 30, 40])
display.plot_cross_hair(5.)
display.set_limits((-40, 40), (-40, 40), ax=ax)
# Radial Velocity
ax = fig.add_subplot(222)
display.plot('VEL_F', 1, ax=ax, cmap = 'pyart_balance')
display.plot_range_rings([10, 20, 30, 40])
display.set_limits((-40, 40), (-40, 40), ax=ax)
#display.set_limits((-300, 300), (-300, 300), ax=ax)
# Corrected Reflectivity - Karen
ax = fig.add_subplot(223)
display.plot('ZM', 1, vmin=-32, vmax=64., ax=ax, cmap = 'pyart_HomeyerRainbow')
display.plot_range_rings([10, 20, 30, 40])
display.set_limits((-40, 40), (-40, 40), ax=ax)
# Corrected Radial Velocity - Karen
ax = fig.add_subplot(224)
display.plot('VM', 1, ax=ax, vmin=-nyq, vmax=nyq, cmap = 'pyart_balance')
display.plot_range_rings([10, 20, 30, 40])
display.set_limits((-40, 40), (-40, 40), ax=ax)
return plt.show();
#plt.savefig('radar_explore/DOW6_comparison.png', dpi=200)
def grid_radars(dow7_file, dow6_file, factor=0.03):
"""
Takes list of radar files and creates a grid
Input
----------
list_files: list of radar files
Output
----------
grid: Pyart Gridded object
"""
# Read in the first file
dow7_radar = pyart.io.read_cfradial(dow7_file)
# Read in the second file
dow6_radar = pyart.io.read_cfradial(dow6_file)
# Create tuple with radars
radars = (dow7_radar, dow6_radar)
# Grab DOW7 latitude and longitude information
grid_lat = dow7_radar.latitude['data'][0]
grid_lon = dow7_radar.longitude['data'][0]
#grid_lat = dow7_radar.latitude['data'][0]
#grid_lon = dow7_radar.longitude['data'][0]
roi = 1500
dow6_grid = pyart.map.grid_from_radars(dow6_radar,
grid_shape=(30, 81, 121),
grid_limits=((500, 15000), (-10000, 30000), (-30000, 30000)),
grid_origin = (grid_lat, grid_lon),
grid_origin_alt = 0,
weighting_function='Barnes2',
#roi_func='dist', xy_factor=factor, z_factor=factor,
roi_func='constant', constant_roi=roi,
fields=['ZM', 'VM'])
dow7_grid = pyart.map.grid_from_radars(dow7_radar,
grid_shape=(30, 81, 121),
grid_limits=((500, 15000), (-10000, 30000), (-30000, 30000)),
grid_origin = (grid_lat, grid_lon),
weighting_function='Barnes2',
grid_origin_alt = 0,
roi_func='constant', constant_roi=roi,
#roi_func='dist', xy_factor=factor, z_factor=factor,
fields=['ZM', 'VM'])
comp_grid = pyart.map.grid_from_radars(radars,
grid_shape=(30, 81, 121),
grid_limits=((500, 15000), (-10000, 30000), (-30000, 30000)),
grid_origin = (grid_lat, grid_lon),
grid_origin_alt = 0,
weighting_function='Barnes2',
#roi_func='dist', xy_factor=factor, z_factor=factor,
roi_func='constant', constant_roi=roi,
fields=['ZM'])
dow7_zm = dow7_grid.fields['ZM']['data']
dow6_zm = dow6_grid.fields['ZM']['data']
comp_zm = comp_grid.fields['ZM']['data']
dow7_vm = dow7_grid.fields['VM']['data']
dow6_vm = dow6_grid.fields['VM']['data']
comp_ma = np.ma.masked_where(np.ma.getmask(dow7_zm), comp_zm)
comp_ma = np.ma.masked_where(np.ma.getmask(dow6_zm), comp_ma)
# Mask based on other field
dow7_vm_ma = np.ma.masked_where(np.ma.getmask(dow6_vm), dow7_vm)
dow6_vm_ma = np.ma.masked_where(np.ma.getmask(dow7_vm), dow6_vm)
comp_grid.fields['ZM']['data'] = comp_ma
dow6_grid.fields['VM']['data'] = dow6_vm_ma
dow7_grid.fields['VM']['data'] = dow7_vm_ma
dow7_grid.add_field('ZM_composite', comp_grid.fields['ZM'])
dow6_grid.add_field('ZM_composite', comp_grid.fields['ZM'])
return dow7_grid, dow6_grid
def dual_doppler_analysis(time, smooth_param=0, use_sounding=True, second_param=0, case='Nov10', radar1='DOW6', radar2='DOW7'):
"""
Runs dual doppler analysis using the specified times
"""
# Read in the files
dow6_files = glob.glob(f'output/{case}/{radar1}/v{time}/*')
dow7_files = glob.glob(f'output/{case}/{radar2}/v{time}/*')
# Read in the sounding
#sounding = pyart.io.read_arm_sonde('soundings/corsondewnpnS1.b1.20181110.180000.custom.cdf')
# Grid the radar data using the function above
dow7_grid, dow6_grid = grid_radars(dow7_files[0], dow6_files[0])
print(list(dow7_grid.fields))
# Make sure only one value is used for each radar lat, lon, and altitude
dow6_grid.radar_latitude['data'] = dow6_grid.radar_latitude['data'][0:1]
dow6_grid.radar_longitude['data'] = dow6_grid.radar_longitude['data'][0:1]
dow6_grid.radar_altitude['data'] = dow6_grid.radar_altitude['data'][0:1]
dow7_grid.origin_altitude['data'] = dow7_grid.origin_altitude['data'][0:1]
dow7_grid.origin_latitude['data'] = dow7_grid.origin_latitude['data'][0:1]
dow7_grid.origin_longitude['data'] = dow7_grid.origin_longitude['data'][0:1]
# Use ERA data to constrain the velocity field intialization
#dow6_grid = pydda.constraints.make_constraint_from_era_interim(dow6_grid, vel_field='VM')
#dow7_grid = pydda.constraints.make_constraint_from_era_interim(dow7_grid, vel_field='VM')
profile_agl = pyart.core.HorizontalWindProfile.from_u_and_v(alt, uwnd, vwnd)
profile_agl.height = profile_agl.height - dow7_grid.origin_altitude['data'][0]
if use_sounding:
# Load sounding data and insert as an intialization
u_init, v_init, w_init = pydda.initialization.make_wind_field_from_profile(dow6_grid, profile_agl, vel_field='VM')
else:
# Otherwise use constant wind field
u_init, v_init, w_init = pydda.initialization.make_constant_wind_field(dow6_grid, vel_field='VM')
#print(list(dow6_grid.fields))
u_back = profile_agl.u_wind[::-1]
v_back = profile_agl.v_wind[::-1]
# Convert to height above ground level relative to the DOW elevation
z_back = profile_agl.height[::-1] #- dow7_grid.origin_altitude['data']
# Run the dual-doppler retrieval
new_grids = pydda.retrieval.get_dd_wind_field([dow6_grid, dow7_grid],
u_init, v_init, w_init, vel_name='VM', refl_field='ZM_composite',
#Co=1, Cm=1500.,
Co=1, Cm=1500,
upper_bc=True,
Cx=second_param, Cy=second_param, #Cz=second_param/10,
#Cb=0.00001, u_back=u_back, v_back=v_back, z_back=z_back,
#model_fields=['erainterim'], Cmod=0.01,
#Cv=1e-2, Ut = 13., Vt = 1.,
#Cmod=1e-3, model_fields=['erainterim'],
mask_outside_opt=True)
# Write the first grid to netcdf containing the dual doppler analysis
new_grids[1].to_xarray().reset_coords().to_netcdf(f'dual_output/{case}/{time}.nc')
###################################
# Plot 6 km Vertical Velocity (w)
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='w', level=12,
show_lobes=True, bg_grid_no=1, vmin=-30, vmax=30,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=20, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='seismic')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'{case} 2018 {time} UTC 6 km /n Smooth Param: {smooth_param}', fontsize=16)
plt.savefig(f'plots/{case}/vert_velocity_{time}_utc_6km.png', dpi=200)
# Close out the figure
plt.close()
##############################
# Plot 3 km Vertical Velocity
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='w', level=6,
show_lobes=True, bg_grid_no=1, vmin=-17, vmax=17,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='seismic')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'{case} 2018 {time} UTC 3 km /n Smooth Param: {smooth_param}', fontsize=16)
plt.savefig(f'plots/{case}/vert_velocity_{time}_utc_3km.png', dpi=200)
plt.close()
#############################
# Plot 3 km Reflectivity
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='ZM_composite', level=6,
show_lobes=True, vmin=-20, vmax=60,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='pyart_HomeyerRainbow')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'{case} 2018 {time} UTC 3 km', fontsize=16)
plt.savefig(f'plots/{case}/reflectivity_{time}_utc_3km.png', dpi=200)
plt.close()
#############################
# Plot 3 km Radial Velocity
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='VM', level=10,
show_lobes=True, bg_grid_no=1, vmin=-30, vmax=30,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='seismic')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'10 November 2018 {time} UTC 3 km', fontsize=16)
plt.savefig(f'plots/vel_{time}_utc_3km.png', dpi=200)
return new_grids
IOP4 10 November Case¶
times = ['1954', '1957', '2001', '2007', '2012', '2014', '2017', '2021']
times = ['2007', '2012', '2014', '2021']
from scipy.interpolate import interp1d
for time in times:
new_grids = dual_doppler_analysis(time, smooth_param=0.5, use_sounding=True, second_param=0.001)
['ZM', 'VM', 'ROI', 'ZM_composite']
Calculating weights for radars 0 and 1
Calculating weights for models...
Starting solver
rmsVR = 19.03594174450314
Total points: 167348
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:408: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:409: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:419: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:420: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] <= math.radians(max_bca))] = 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca))] = 1
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2385.7804| 563.8219|3011.2799| 0.0000| 0.0000| 0.0000| 0.0000| 24.4185
Norm of gradient: 0.26965198969701404
Iterations before filter: 10
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1181.4085| 346.3609|2070.6446| 0.0000| 0.0000| 0.0000| 0.0000| 23.4440
Norm of gradient: 0.2056178922401188
Iterations before filter: 20
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 896.4463| 317.1363|1700.7667| 0.0000| 0.0000| 0.0000| 0.0000| 23.7292
Norm of gradient: 0.09270840757062238
Iterations before filter: 30
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 789.1178| 266.9600|1503.2391| 0.0000| 0.0000| 0.0000| 0.0000| 23.7720
Norm of gradient: 0.07145387099594375
Iterations before filter: 40
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 716.7098| 260.5924|1390.5651| 0.0000| 0.0000| 0.0000| 0.0000| 23.9526
Norm of gradient: 0.027847728649060884
Iterations before filter: 50
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 663.8567| 236.9330|1330.7616| 0.0000| 0.0000| 0.0000| 0.0000| 23.6810
Norm of gradient: 0.04970064559051249
Iterations before filter: 60
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 632.2378| 230.3709|1267.0442| 0.0000| 0.0000| 0.0000| 0.0000| 23.6778
Norm of gradient: 0.05032325372251866
Iterations before filter: 70
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 620.4242| 214.5341|1211.2242| 0.0000| 0.0000| 0.0000| 0.0000| 23.3791
Norm of gradient: 0.017713028436688833
Iterations before filter: 80
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 602.7136| 211.9196|1170.8601| 0.0000| 0.0000| 0.0000| 0.0000| 23.3737
Norm of gradient: 0.023094404881194068
Iterations before filter: 90
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 597.3454| 200.7072|1137.8073| 0.0000| 0.0000| 0.0000| 0.0000| 23.1141
Norm of gradient: 0.0063613651660078905
Iterations before filter: 100
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 579.3498| 199.1999|1116.3079| 0.0000| 0.0000| 0.0000| 0.0000| 23.0791
Norm of gradient: 0.015206142832890027
Iterations before filter: 110
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 585.8276| 189.2797|1088.8775| 0.0000| 0.0000| 0.0000| 0.0000| 22.7895
Norm of gradient: 0.011947512369457331
Iterations before filter: 120
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 568.8100| 189.9797|1072.1477| 0.0000| 0.0000| 0.0000| 0.0000| 22.7839
Norm of gradient: 0.014086234869916888
Iterations before filter: 130
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 566.2892| 181.9888|1052.9029| 0.0000| 0.0000| 0.0000| 0.0000| 22.5281
Norm of gradient: 0.011518091125647275
Iterations before filter: 140
Applying low pass filter to wind field...
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1200.7276| 137.6723| 979.8800| 0.0000| 0.0000| 0.0000| 0.0000| 15.4163
Norm of gradient: 0.1428517733483083
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1189.5929| 137.7142| 975.0434| 0.0000| 0.0000| 0.0000| 0.0000| 15.4377
Norm of gradient: 0.12512514894422816
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 766.3067| 165.8939|1062.1570| 0.0000| 0.0000| 0.0000| 0.0000| 17.2217
Norm of gradient: 0.44617253672092316
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 654.0598| 184.3512|1334.7118| 0.0000| 0.0000| 0.0000| 0.0000| 18.3592
Norm of gradient: 0.7716539804507561
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 720.7249| 170.0813|1029.5911| 0.0000| 0.0000| 0.0000| 0.0000| 17.6200
Norm of gradient: 0.10717367157183733
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 741.9438| 164.0900| 987.6000| 0.0000| 0.0000| 0.0000| 0.0000| 17.5058
Norm of gradient: 0.08143157305612776
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 721.1884| 164.2323| 979.3928| 0.0000| 0.0000| 0.0000| 0.0000| 17.8581
Norm of gradient: 0.061792056889757266
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 643.1934| 176.6913|1024.1694| 0.0000| 0.0000| 0.0000| 0.0000| 19.0394
Norm of gradient: 0.11598064089086615
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 624.6354| 180.5768|1020.4126| 0.0000| 0.0000| 0.0000| 0.0000| 19.3466
Norm of gradient: 0.038800631262407695
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 613.6000| 182.8277|1024.0315| 0.0000| 0.0000| 0.0000| 0.0000| 19.5347
Norm of gradient: 0.022732182461436382
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 596.7959| 186.1320|1030.9836| 0.0000| 0.0000| 0.0000| 0.0000| 19.9045
Norm of gradient: 0.041083610262878906
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 576.7002| 190.1544|1040.8888| 0.0000| 0.0000| 0.0000| 0.0000| 20.5545
Norm of gradient: 0.04459946235241034
Iterations after filter: 1
Iterations after filter: 2
Done! Time = 87.7
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
['ZM', 'VM', 'ROI', 'ZM_composite']
Calculating weights for radars 0 and 1
Calculating weights for models...
Starting solver
rmsVR = 15.114126801162826
Total points: 142166
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:408: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:409: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:419: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:420: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] <= math.radians(max_bca))] = 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca))] = 1
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2660.2645| 565.0598|3533.4481| 0.0000| 0.0000| 0.0000| 0.0000| 33.9547
Norm of gradient: 0.1378636703981757
Iterations before filter: 10
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1118.9704| 386.6984|2466.7303| 0.0000| 0.0000| 0.0000| 0.0000| 31.5961
Norm of gradient: 0.10130368903703041
Iterations before filter: 20
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 949.9282| 317.3620|1942.9976| 0.0000| 0.0000| 0.0000| 0.0000| 33.7857
Norm of gradient: 0.03370152004001552
Iterations before filter: 30
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 860.8240| 272.4921|1756.6272| 0.0000| 0.0000| 0.0000| 0.0000| 32.4604
Norm of gradient: 0.03590783323620595
Iterations before filter: 40
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 782.7759| 252.9373|1632.4298| 0.0000| 0.0000| 0.0000| 0.0000| 34.6054
Norm of gradient: 0.044452682235541545
Iterations before filter: 50
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 759.8727| 231.0059|1544.2291| 0.0000| 0.0000| 0.0000| 0.0000| 33.5941
Norm of gradient: 0.06889431241931993
Iterations before filter: 60
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 729.1434| 225.5021|1473.6133| 0.0000| 0.0000| 0.0000| 0.0000| 35.0392
Norm of gradient: 0.04044140410559862
Iterations before filter: 70
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 722.4413| 210.6913|1413.3714| 0.0000| 0.0000| 0.0000| 0.0000| 34.3624
Norm of gradient: 0.020879348492745357
Iterations before filter: 80
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 700.5925| 207.6170|1374.4724| 0.0000| 0.0000| 0.0000| 0.0000| 35.5806
Norm of gradient: 0.020770671204342023
Iterations before filter: 90
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 695.4625| 197.9551|1340.6321| 0.0000| 0.0000| 0.0000| 0.0000| 34.9556
Norm of gradient: 0.008006754772193567
Iterations before filter: 100
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 682.3125| 195.7283|1310.1148| 0.0000| 0.0000| 0.0000| 0.0000| 35.9546
Norm of gradient: 0.011204301682504083
Iterations before filter: 110
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 680.4659| 188.1285|1284.7154| 0.0000| 0.0000| 0.0000| 0.0000| 35.3193
Norm of gradient: 0.006399805443678243
Iterations before filter: 120
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 667.1409| 183.9229|1264.2836| 0.0000| 0.0000| 0.0000| 0.0000| 35.8136
Norm of gradient: 0.01557917522863525
Iterations before filter: 130
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 666.8705| 179.0928|1227.6108| 0.0000| 0.0000| 0.0000| 0.0000| 35.8747
Norm of gradient: 0.039284041303490946
Iterations before filter: 140
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 654.6403| 175.1364|1208.6149| 0.0000| 0.0000| 0.0000| 0.0000| 36.0915
Norm of gradient: 0.008847126284513014
Iterations before filter: 150
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 657.2883| 170.3108|1183.0131| 0.0000| 0.0000| 0.0000| 0.0000| 35.9987
Norm of gradient: 0.01859422921258853
Iterations before filter: 160
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 644.5606| 168.0423|1171.9550| 0.0000| 0.0000| 0.0000| 0.0000| 36.2549
Norm of gradient: 0.006872963286791082
Iterations before filter: 170
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 652.2107| 161.6995|1135.1166| 0.0000| 0.0000| 0.0000| 0.0000| 36.0821
Norm of gradient: 0.016204911703462357
Iterations before filter: 180
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 634.0205| 159.2911|1127.1341| 0.0000| 0.0000| 0.0000| 0.0000| 36.4078
Norm of gradient: 0.004024582168366484
Iterations before filter: 190
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 635.2694| 155.5699|1097.5382| 0.0000| 0.0000| 0.0000| 0.0000| 36.5954
Norm of gradient: 0.013818497911772918
Iterations before filter: 200
Applying low pass filter to wind field...
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1654.2579| 89.7056| 996.0122| 0.0000| 0.0000| 0.0000| 0.0000| 20.3311
Norm of gradient: 0.2127773186755198
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1629.2807| 89.7321| 988.9909| 0.0000| 0.0000| 0.0000| 0.0000| 20.3428
Norm of gradient: 0.209903953040575
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 911.1799| 131.6864|1113.6528| 0.0000| 0.0000| 0.0000| 0.0000| 25.7405
Norm of gradient: 0.37286245910682675
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 753.3706| 160.6752|1580.3824| 0.0000| 0.0000| 0.0000| 0.0000| 30.6181
Norm of gradient: 0.7989236912916559
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 846.5253| 137.6900|1071.9508| 0.0000| 0.0000| 0.0000| 0.0000| 27.3158
Norm of gradient: 0.10784046394826906
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 862.5901| 130.5764|1026.8507| 0.0000| 0.0000| 0.0000| 0.0000| 27.0297
Norm of gradient: 0.07986213503179665
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 829.3796| 130.4495|1016.0622| 0.0000| 0.0000| 0.0000| 0.0000| 28.4707
Norm of gradient: 0.06221117802586161
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 727.5910| 147.0902|1080.3474| 0.0000| 0.0000| 0.0000| 0.0000| 32.6543
Norm of gradient: 0.12154829550316731
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 711.1430| 150.2747|1065.7925| 0.0000| 0.0000| 0.0000| 0.0000| 33.2876
Norm of gradient: 0.035709100023258944
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 696.5285| 153.2016|1069.8960| 0.0000| 0.0000| 0.0000| 0.0000| 33.8928
Norm of gradient: 0.026858419157479593
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 674.1206| 157.6951|1077.6219| 0.0000| 0.0000| 0.0000| 0.0000| 35.0192
Norm of gradient: 0.03230576187821094
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 655.5862| 161.8357|1081.6356| 0.0000| 0.0000| 0.0000| 0.0000| 36.4056
Norm of gradient: 0.040760719296840775
Iterations after filter: 1
Iterations after filter: 2
Done! Time = 113.7
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
['ZM', 'VM', 'ROI', 'ZM_composite']
Calculating weights for radars 0 and 1
Calculating weights for models...
Starting solver
rmsVR = 13.496556932974375
Total points: 130276
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:408: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:409: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:419: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:420: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] <= math.radians(max_bca))] = 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca))] = 1
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2348.8622| 742.5180|4072.3844| 0.0000| 0.0000| 0.0000| 0.0000| 29.6148
Norm of gradient: 0.16394739941319164
Iterations before filter: 10
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1215.4138| 457.1836|2656.1494| 0.0000| 0.0000| 0.0000| 0.0000| 28.1556
Norm of gradient: 0.05811384888038545
Iterations before filter: 20
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 962.6794| 380.8780|2266.2197| 0.0000| 0.0000| 0.0000| 0.0000| 32.4424
Norm of gradient: 0.21080547319561793
Iterations before filter: 30
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 871.9432| 320.2638|1908.1383| 0.0000| 0.0000| 0.0000| 0.0000| 30.9040
Norm of gradient: 0.02147858357243421
Iterations before filter: 40
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 810.1526| 302.4323|1771.1405| 0.0000| 0.0000| 0.0000| 0.0000| 32.7816
Norm of gradient: 0.023068403350740427
Iterations before filter: 50
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 789.8849| 269.1216|1672.4541| 0.0000| 0.0000| 0.0000| 0.0000| 31.9280
Norm of gradient: 0.030279090860364295
Iterations before filter: 60
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 746.2419| 260.9243|1622.3280| 0.0000| 0.0000| 0.0000| 0.0000| 33.3533
Norm of gradient: 0.05092919543016084
Iterations before filter: 70
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 754.1833| 241.0865|1543.4696| 0.0000| 0.0000| 0.0000| 0.0000| 32.9423
Norm of gradient: 0.015663634036932675
Iterations before filter: 80
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 729.3557| 237.9571|1503.8305| 0.0000| 0.0000| 0.0000| 0.0000| 34.1102
Norm of gradient: 0.019107812774741623
Iterations before filter: 90
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 731.2199| 224.9792|1466.2241| 0.0000| 0.0000| 0.0000| 0.0000| 33.7108
Norm of gradient: 0.008897447584644728
Iterations before filter: 100
Applying low pass filter to wind field...
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2122.0540| 146.9797|1396.3226| 0.0000| 0.0000| 0.0000| 0.0000| 24.9075
Norm of gradient: 0.2904910461608383
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2080.4643| 147.0437|1386.2431| 0.0000| 0.0000| 0.0000| 0.0000| 24.9345
Norm of gradient: 0.2674584229250857
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1050.0424| 201.4075|1537.4854| 0.0000| 0.0000| 0.0000| 0.0000| 26.1567
Norm of gradient: 0.289383348563477
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 858.3126| 237.0235|2179.7708| 0.0000| 0.0000| 0.0000| 0.0000| 27.8748
Norm of gradient: 0.7664430992523129
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 968.0289| 207.9678|1479.1873| 0.0000| 0.0000| 0.0000| 0.0000| 26.6696
Norm of gradient: 0.11669631526235033
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 982.4417| 199.8295|1425.5064| 0.0000| 0.0000| 0.0000| 0.0000| 26.6338
Norm of gradient: 0.07911152077737238
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 940.0794| 199.5582|1405.8623| 0.0000| 0.0000| 0.0000| 0.0000| 27.2158
Norm of gradient: 0.060380738323319916
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 819.2467| 221.0171|1478.4367| 0.0000| 0.0000| 0.0000| 0.0000| 28.7322
Norm of gradient: 0.1569248250858718
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 799.3745| 224.7627|1455.5369| 0.0000| 0.0000| 0.0000| 0.0000| 28.9472
Norm of gradient: 0.05054362337466886
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 780.7967| 228.6284|1458.8744| 0.0000| 0.0000| 0.0000| 0.0000| 29.1820
Norm of gradient: 0.03323622289657599
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 755.4370| 234.6121|1463.6163| 0.0000| 0.0000| 0.0000| 0.0000| 29.6081
Norm of gradient: 0.03352764783680135
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 739.0881| 240.1346|1458.6793| 0.0000| 0.0000| 0.0000| 0.0000| 30.0905
Norm of gradient: 0.04168860539869632
Iterations after filter: 1
Iterations after filter: 2
Done! Time = 67.6
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
['ZM', 'VM', 'ROI', 'ZM_composite']
Calculating weights for radars 0 and 1
Calculating weights for models...
Starting solver
rmsVR = 15.404588782409398
Total points: 144184
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:408: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:409: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:419: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:420: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] <= math.radians(max_bca))] = 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca))] = 1
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|4191.1339| 881.0547|4223.5028| 0.0000| 0.0000| 0.0000| 0.0000| 30.8509
Norm of gradient: 0.1256154879734669
Iterations before filter: 10
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2070.3606| 685.2602|3042.8861| 0.0000| 0.0000| 0.0000| 0.0000| 25.7752
Norm of gradient: 0.04792967940402924
Iterations before filter: 20
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1620.2912| 579.3535|2563.0267| 0.0000| 0.0000| 0.0000| 0.0000| 26.9963
Norm of gradient: 0.061709362199629664
Iterations before filter: 30
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1547.6806| 485.1756|2370.0348| 0.0000| 0.0000| 0.0000| 0.0000| 25.3269
Norm of gradient: 0.08645502175009437
Iterations before filter: 40
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1432.3378| 457.6403|2240.4885| 0.0000| 0.0000| 0.0000| 0.0000| 26.2232
Norm of gradient: 0.04855832842610265
Iterations before filter: 50
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1452.7172| 394.7777|2097.7443| 0.0000| 0.0000| 0.0000| 0.0000| 26.4830
Norm of gradient: 0.031458551474678
Iterations before filter: 60
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1354.3945| 375.3238|2051.9142| 0.0000| 0.0000| 0.0000| 0.0000| 27.0166
Norm of gradient: 0.023949723195245097
Iterations before filter: 70
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1339.8107| 340.2173|1978.1810| 0.0000| 0.0000| 0.0000| 0.0000| 29.3041
Norm of gradient: 0.07107508314872382
Iterations before filter: 80
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1306.7548| 323.3018|1925.1992| 0.0000| 0.0000| 0.0000| 0.0000| 29.5808
Norm of gradient: 0.022787764622197962
Iterations before filter: 90
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1309.2749| 294.5999|1862.3249| 0.0000| 0.0000| 0.0000| 0.0000| 31.0719
Norm of gradient: 0.020525627000723405
Iterations before filter: 100
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1280.7965| 287.1073|1844.9521| 0.0000| 0.0000| 0.0000| 0.0000| 31.2436
Norm of gradient: 0.01236608856213324
Iterations before filter: 110
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1293.0073| 262.4049|1793.2551| 0.0000| 0.0000| 0.0000| 0.0000| 32.3819
Norm of gradient: 0.015513330589888519
Iterations before filter: 120
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1260.6391| 257.9080|1782.1509| 0.0000| 0.0000| 0.0000| 0.0000| 32.4921
Norm of gradient: 0.005769090353404301
Iterations before filter: 130
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1273.7381| 240.3974|1742.6648| 0.0000| 0.0000| 0.0000| 0.0000| 33.1043
Norm of gradient: 0.011918077115545607
Iterations before filter: 140
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1248.8074| 237.8157|1735.2304| 0.0000| 0.0000| 0.0000| 0.0000| 33.1750
Norm of gradient: 0.006652549590620723
Iterations before filter: 150
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1254.9401| 231.3909|1720.8211| 0.0000| 0.0000| 0.0000| 0.0000| 33.2990
Norm of gradient: 0.016365352005926773
Iterations before filter: 160
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1243.9046| 230.5602|1718.3368| 0.0000| 0.0000| 0.0000| 0.0000| 33.3484
Norm of gradient: 0.002666858179271438
Iterations before filter: 170
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1250.5334| 223.7709|1701.3022| 0.0000| 0.0000| 0.0000| 0.0000| 33.4584
Norm of gradient: 0.0137201611486545
Iterations before filter: 180
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1240.5683| 223.3843|1699.3664| 0.0000| 0.0000| 0.0000| 0.0000| 33.4913
Norm of gradient: 0.0021068553840788563
Iterations before filter: 190
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1243.7845| 215.6400|1687.4606| 0.0000| 0.0000| 0.0000| 0.0000| 33.5896
Norm of gradient: 0.01620072171787526
Iterations before filter: 200
Applying low pass filter to wind field...
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2582.4272| 141.2081|1659.6692| 0.0000| 0.0000| 0.0000| 0.0000| 31.7632
Norm of gradient: 0.22909408797832714
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|2550.4785| 140.9991|1642.6785| 0.0000| 0.0000| 0.0000| 0.0000| 31.7609
Norm of gradient: 0.21197052186375998
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1711.6917| 188.1008|1659.2943| 0.0000| 0.0000| 0.0000| 0.0000| 31.6190
Norm of gradient: 0.4711054388356092
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1481.8021| 220.0327|2267.6799| 0.0000| 0.0000| 0.0000| 0.0000| 31.5315
Norm of gradient: 0.9151327129202761
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1612.5988| 194.3738|1590.1617| 0.0000| 0.0000| 0.0000| 0.0000| 31.5887
Norm of gradient: 0.14066595086587347
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1614.6862| 183.4431|1545.0922| 0.0000| 0.0000| 0.0000| 0.0000| 31.5918
Norm of gradient: 0.07087261978240864
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1537.7328| 183.1647|1570.5543| 0.0000| 0.0000| 0.0000| 0.0000| 31.5638
Norm of gradient: 0.06845955879262619
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1355.9944| 202.0002|1693.8886| 0.0000| 0.0000| 0.0000| 0.0000| 31.4914
Norm of gradient: 0.1343425471597947
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1325.1426| 208.3546|1687.4030| 0.0000| 0.0000| 0.0000| 0.0000| 31.4727
Norm of gradient: 0.04504796132573377
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1315.9811| 211.4567|1683.6636| 0.0000| 0.0000| 0.0000| 0.0000| 31.4636
Norm of gradient: 0.03311283239367466
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1298.2359| 217.0663|1682.6580| 0.0000| 0.0000| 0.0000| 0.0000| 31.4407
Norm of gradient: 0.048951364921909934
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|1280.3195| 225.4955|1682.8383| 0.0000| 0.0000| 0.0000| 0.0000| 31.3845
Norm of gradient: 0.06556345531480742
Iterations after filter: 1
Iterations after filter: 2
Done! Time = 111.9
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
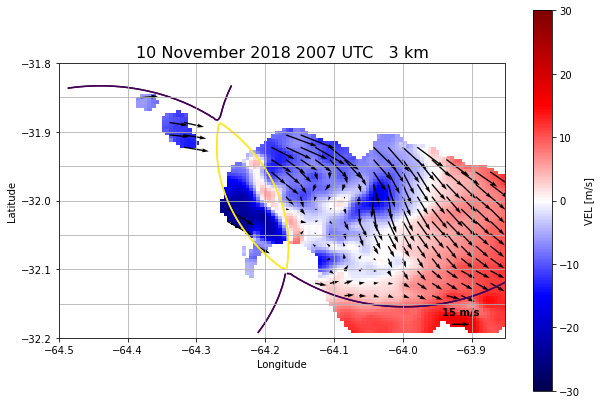
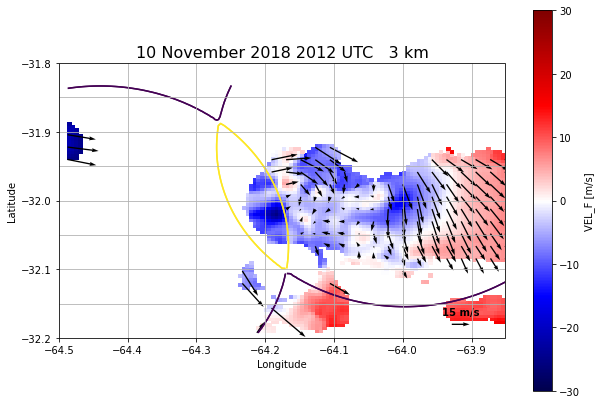
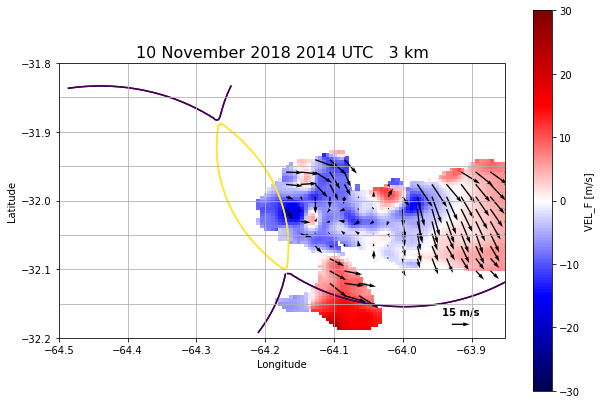
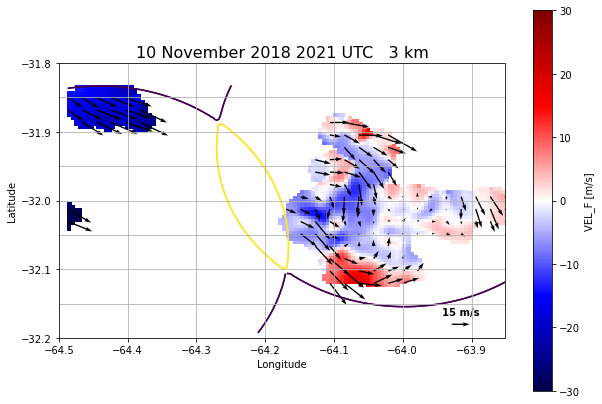
dow6_grid, dow7_grid = new_grids
dow7_dda = new_grids[1].to_xarray().squeeze()
dow6_dda = new_grids[0].to_xarray().squeeze()
dow7_dda = dow7_dda.where((dow7_dda.ZM > -10) & (dow6_dda.ZM > -10))
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='ZM', level=3,
show_lobes=True, vmin=-20, vmax=60,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='pyart_HomeyerRainbow')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
#plt.title(f'{case} 2018 {time} UTC 3 km', fontsize=16)
#plt.savefig(f'plots/{case}/reflectivity_{time}_utc_3km.png', dpi=200)
plt.show()
plt.close()
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
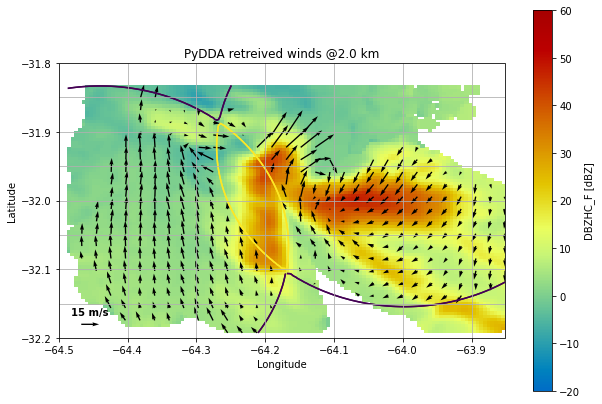
plt.figure(figsize=(10,8))
dow7_dda.sel(z=5000).ZM.plot(vmin=-20, vmax=60, cmap='pyart_HomeyerRainbow')
<matplotlib.collections.QuadMesh at 0x7fe584ffbed0>
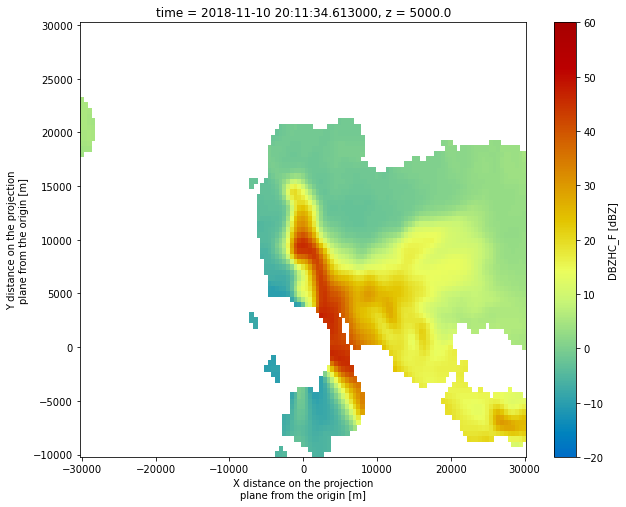
plt.figure(figsize=(12,8))
dow7_dda.sel(y=14000).ZM_composite.plot(vmin=-20, vmax=60, cmap='pyart_HomeyerRainbow')
dow7_dda.where(dow7_dda.w>1).sel(y=14000).w.plot.contourf(cmap='Reds')
dow7_dda.where(dow7_dda.w<-3).sel(y=14000).w.plot.contourf(cmap='Blues_r')
<matplotlib.contour.QuadContourSet at 0x7fe58524c090>
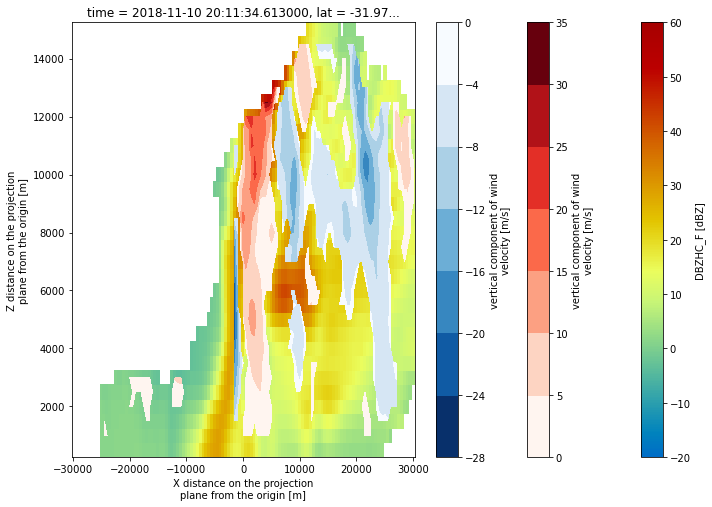
dow6_dda.sel(z=5000).ZM_composite.plot(vmin=-20, vmax=60, cmap='pyart_HomeyerRainbow')
<matplotlib.collections.QuadMesh at 0x7fae81d9bc10>
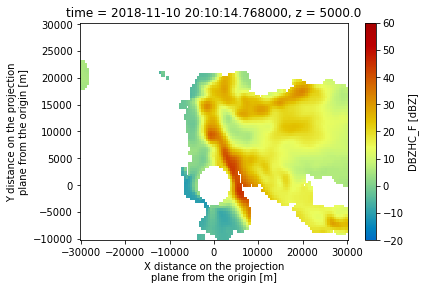
dow6_dda.sel(z=5000).w.plot()
plt.axhline(14000)
<matplotlib.lines.Line2D at 0x7fae84390b50>
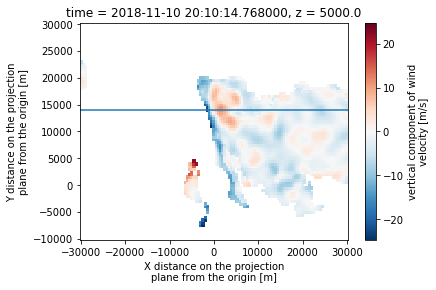
dow7_dda.sel(x=0).VM.plot()
<matplotlib.collections.QuadMesh at 0x7fae7fef7310>
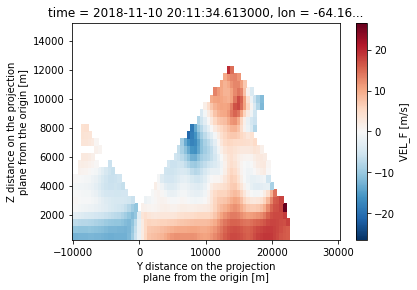
dow7_dda.sel(x=0).w.plot()
<matplotlib.collections.QuadMesh at 0x7fae80594910>
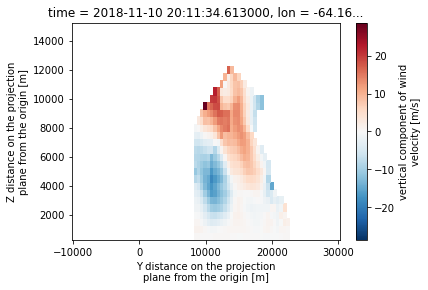
dow6_dda.sel(x=0).ZM_composite.plot(cmap='pyart_HomeyerRainbow', vmin=-20, vmax=70)
<matplotlib.collections.QuadMesh at 0x7fae7c4e5a90>
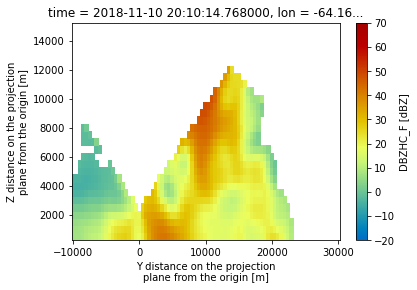
dow6_dda.sel(y=3000).ZM_composite.plot(vmin=-20, vmax=60, cmap='pyart_HomeyerRainbow')
plt.ylim(0,12000)
(0.0, 12000.0)
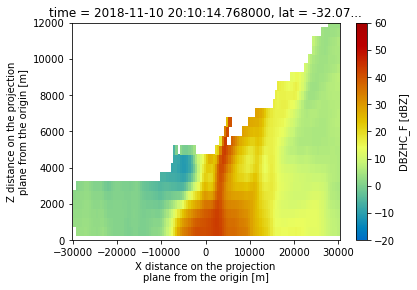
plt.figure(figsize=(12,8))
dow7_dda.sel(y=15000).ZM_composite.plot(vmin=-20, vmax=70, cmap='pyart_HomeyerRainbow')
dow7_dda.sel(y=15000).w.plot(cmap='coolwarm', vmin=-30, vmax=30)
<matplotlib.collections.QuadMesh at 0x7fae80af1410>
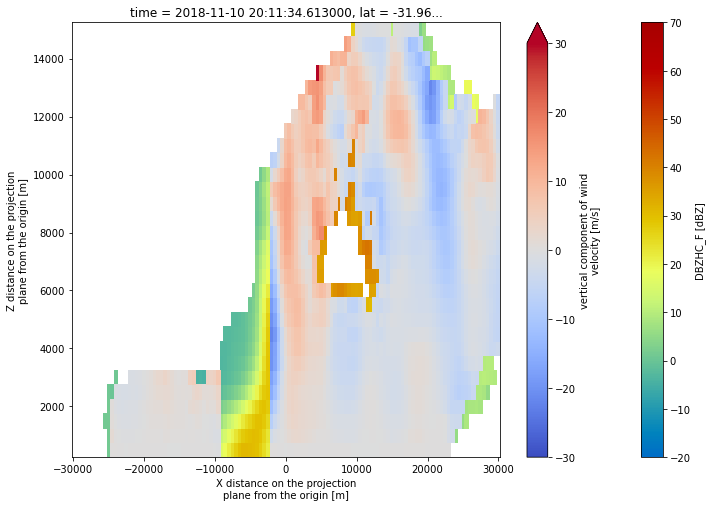
dow7_dda.sel(z=10000).w.plot()
<matplotlib.collections.QuadMesh at 0x7fae7ff72f10>
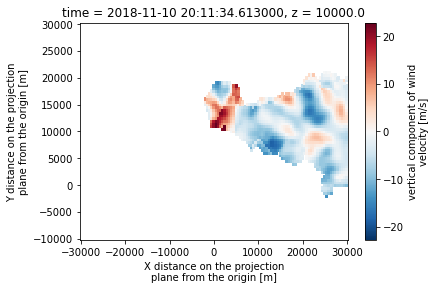
dow7_dda.sel(y=12000).ZM_composite.plot(vmin=-20, vmax=60, cmap='pyart_HomeyerRainbow')
dow6_dda.sel(y=12000).v.plot(cmap='coolwarm')
<matplotlib.collections.QuadMesh at 0x7fa84e2c3110>
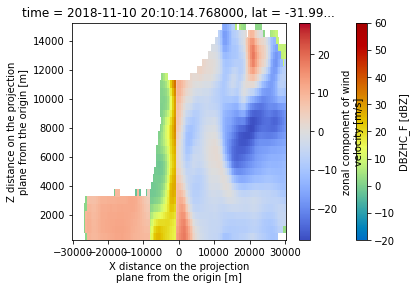
dow6_dda.sel(y=3000).w.plot()
plt.ylim(0,5000)
(0.0, 5000.0)
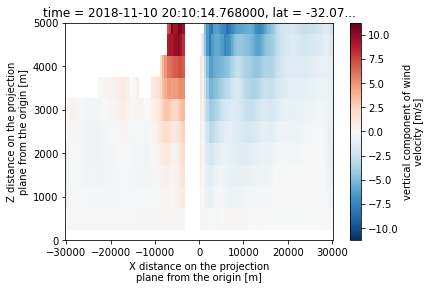
dow7_dda.sel(y=12500).v.plot()
<matplotlib.collections.QuadMesh at 0x7fa837c38490>
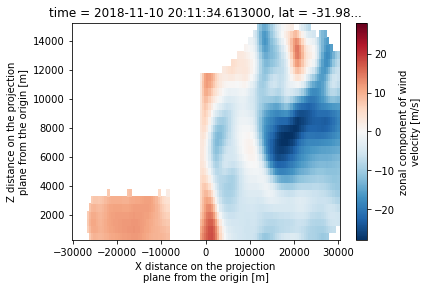
dow7_dda.sel(y=12500).v.plot()
<matplotlib.collections.QuadMesh at 0x7fa8467c2d50>
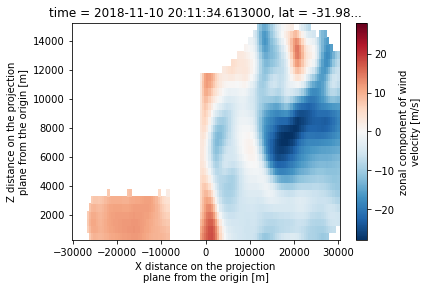
dow7_dda.sel(z=3000).w.plot(vmin=-30, vmax=30, cmap='seismic')
<matplotlib.collections.QuadMesh at 0x7fa83192f890>
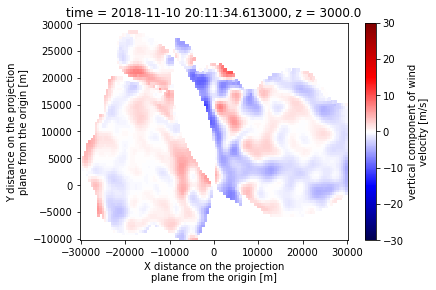
dow7_dda.sel(z=3000).ZM_composite.plot(vmin=-20, vmax=60, cmap='pyart_HomeyerRainbow')
<matplotlib.collections.QuadMesh at 0x7fa82604da10>
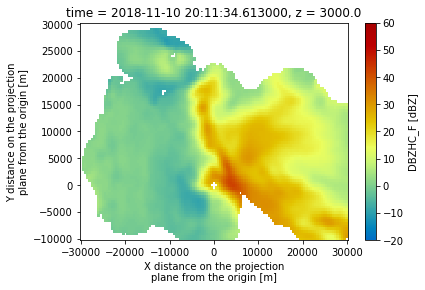
dow7_dda.sel(y=12500).v.plot()
<matplotlib.collections.QuadMesh at 0x7fa838d3b210>
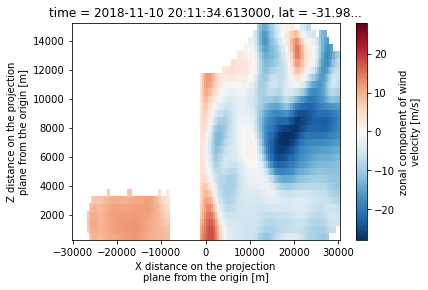
grid.sel(y=5000).ZM.plot(vmin=-20, vmax=70, cmap='pyart_HomeyerRainbow')
<matplotlib.collections.QuadMesh at 0x7fa82a322c10>
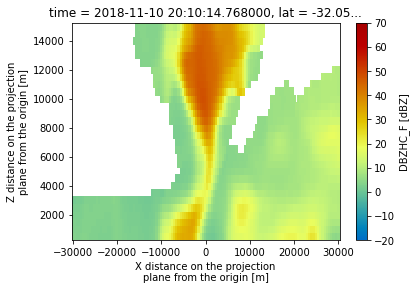
(profile.height[::-1] - new_grids[0].radar_altitude['data'][0])[3]
41.80000667572017
fig = plt.figure(figsize=(10,6))
pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='ZM_composite', level=5,
show_lobes=True, bg_grid_no=1, vmin=-20, vmax=70,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='pyart_HomeyerRainbow')
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
<cartopy.mpl.geoaxes.GeoAxesSubplot at 0x7fa82c3182d0>
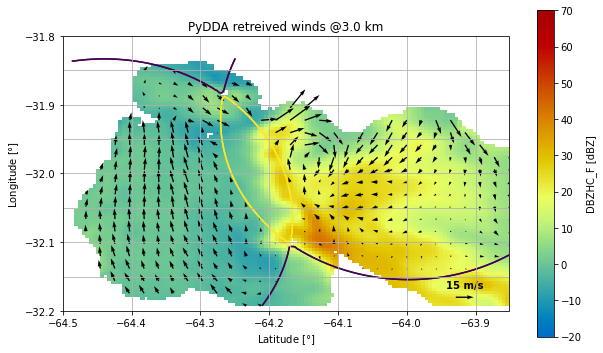
new_grids[0].point_altitude['data'][0]
array([[500., 500., 500., ..., 500., 500., 500.],
[500., 500., 500., ..., 500., 500., 500.],
[500., 500., 500., ..., 500., 500., 500.],
...,
[500., 500., 500., ..., 500., 500., 500.],
[500., 500., 500., ..., 500., 500., 500.],
[500., 500., 500., ..., 500., 500., 500.]])
# Plot a vertical X-Z cross section
plt.figure(figsize=(9, 9))
pydda.vis.plot_horiz_xsection_quiver(new_grids, background_field='ZM_composite',
level=10, w_vel_contours=np.arange(1, 26, 3),
quiverkey_len=10,
vmin=-20, vmax=60, quiver_width=0.005,
cmap='pyart_HomeyerRainbow')
plt.show()
/Users/mgrover/git_repos/PyDDA/pydda/vis/quiver_plot.py:213: UserWarning: linewidths is ignored by contourf
alpha=contour_alpha)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/git_repos/PyDDA/pydda/vis/quiver_plot.py:247: UserWarning: The following kwargs were not used by contour: 'color'
levels=[bca_min, bca_max], color='k')
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/git_repos/PyDDA/pydda/vis/quiver_plot.py:247: UserWarning: The following kwargs were not used by contour: 'color'
levels=[bca_min, bca_max], color='k')
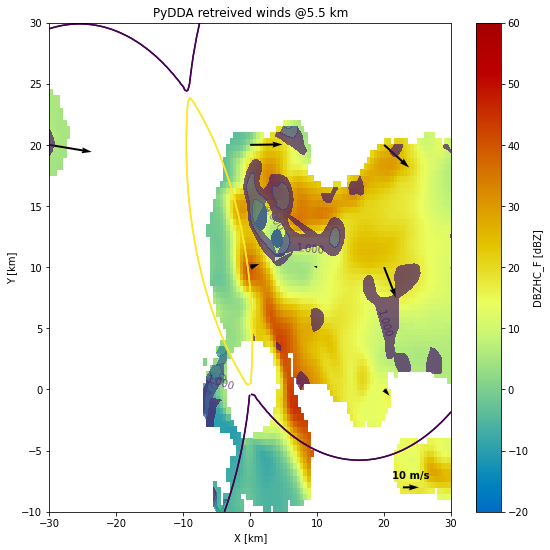
# Plot a vertical X-Z cross section
plt.figure(figsize=(9, 9))
pydda.vis.plot_xz_xsection_quiver(new_grids, background_field='ZM_composite',
level=45,
w_vel_contours=np.arange(2, 50, 4),
quiver_spacing_x_km=2.5,
quiver_spacing_z_km=.5,
quiverkey_len=10,
vmin=-20, vmax=60, quiver_width=0.005,
cmap='pyart_HomeyerRainbow')
plt.ylim(0, 12)
plt.show()
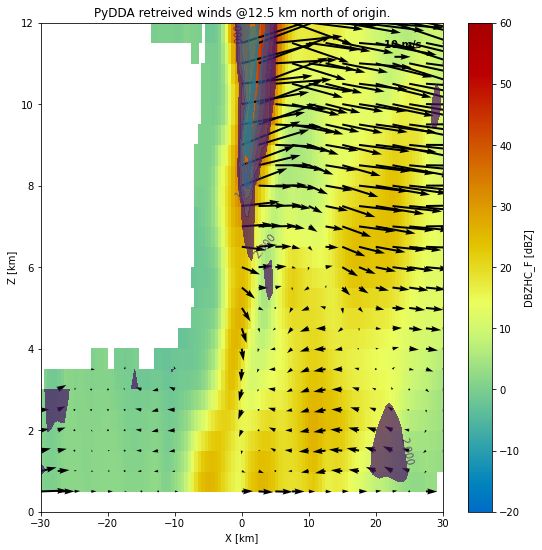
# Plot a vertical X-Z cross section
plt.figure(figsize=(9, 9))
pydda.vis.plot_xz_xsection_quiver(new_grids, background_field='ZM_composite',
level=45,
w_vel_contours=np.arange(-40, 0, 4),
quiver_spacing_x_km=2.5,
quiver_spacing_z_km=.5,
quiverkey_len=5,
vmin=-20, vmax=60, quiver_width=0.005,
cmap='pyart_HomeyerRainbow')
plt.ylim(0, 6)
plt.show()
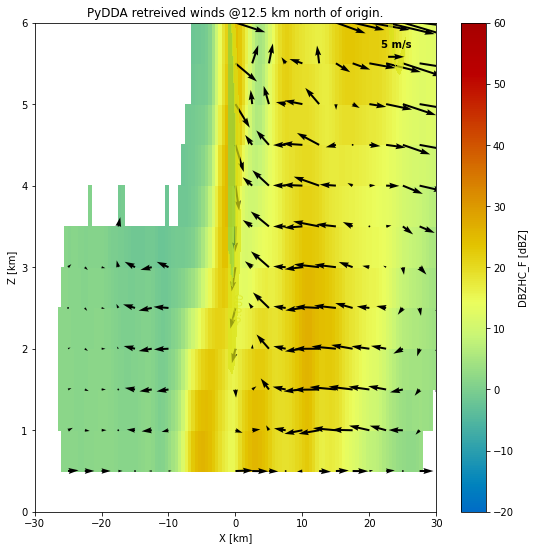
grid = new_grids[0].to_xarray().squeeze()
grid.sel(z=13000).w.plot()
<matplotlib.collections.QuadMesh at 0x7fa832aee490>
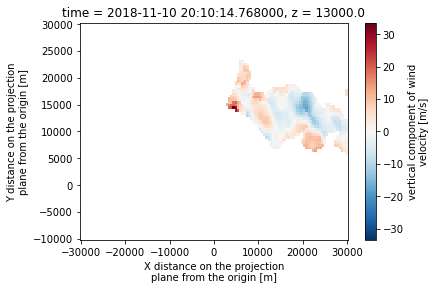
grid.sel(z=6000).ZM_composite.plot()
<matplotlib.collections.QuadMesh at 0x7fa83339c590>
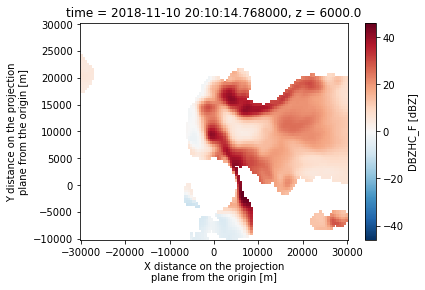
grid.sel(y=12500).v.plot()
<matplotlib.collections.QuadMesh at 0x7fa835c3b190>
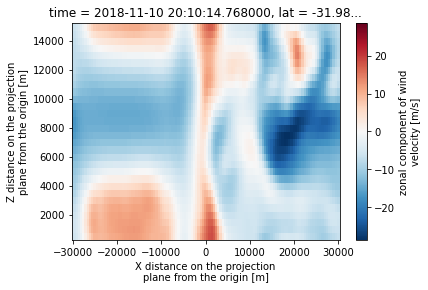
lev = 7000
grid.sel(z=lev).ZM_composite.plot(cmap='Greys')
grid.where(grid.w > 5).sel(z=lev).w.plot(cmap='Reds')
grid.where(grid.w < -5).sel(z=lev).w.plot(cmap='Blues')
<matplotlib.collections.QuadMesh at 0x7fa836024050>
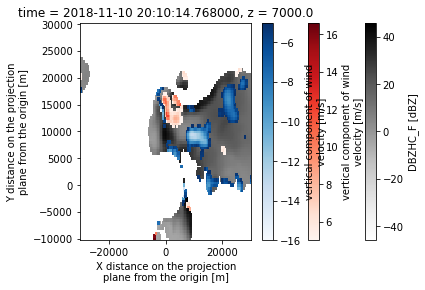
for time in times:
dual_doppler_analysis(time, smooth_param=0.5, use_sounding=True, second_param=0.01)
['ZM', 'VM', 'ROI', 'ZM_composite']
Interpolating sounding to radar grid
Interpolated U field:
[ 0.61185031 -1.47755905 -3.11542971 1.27490636 0.98365897 4.59610825
11.56590905 13.22328765 14.42246695 16.88097903 16.26198126 15.486934
24.26524903 29.94361949 33.6408602 33.91002067 33.26630542 34.42432932
35.36828193 40.26716417 41.88335986 43.37174393 45.39279215 49.89085485
51.89810168 51.09769665 40.63088394 38.73612332 36.24062356 37.56390412
27.50475448]
Interpolated V field:
[ -5.26070678 -8.5 -7.74726563 -7.42471909 -1.2941386
1.26006765 -0.84951296 -8.46149163 -9.87958884 -9.80951048
-9.63306558 -8.92690818 -3.74969622 -8.04733177 -9.4311828
-11.65330578 -14.23044334 -16.79278447 -14.92537446 -12.47014927
-10.19522294 -9.81883738 -8.08481457 -7.78336648 -7.81769745
-9.82140454 0.88509051 -4.98340675 -0.51205871 3.52406392
4.50007798]
Grid levels:
[ 0. 500. 1000. 1500. 2000. 2500. 3000. 3500. 4000. 4500.
5000. 5500. 6000. 6500. 7000. 7500. 8000. 8500. 9000. 9500.
10000. 10500. 11000. 11500. 12000. 12500. 13000. 13500. 14000. 14500.
15000.]
Calculating weights for radars 0 and 1
Calculating weights for models...
Starting solver
rmsVR = 20.748899638557944
Total points: 203787
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:408: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:409: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:419: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:420: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] <= math.radians(max_bca))] = 1
/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca))] = 1
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|31571.3042| 33.6316|20742.5778| 31.5501| 0.0000| 0.0000| 0.0000| 5.7617
Norm of gradient: 1.48853292906004
Iterations before filter: 10
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|15573.0582| 262.7996|13843.3908| 70.1656| 0.0000| 0.0000| 0.0000| 16.9809
Norm of gradient: 0.47370527232067056
Iterations before filter: 20
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|13592.2172| 330.3698|9118.7278| 78.0776| 0.0000| 0.0000| 0.0000| 19.5098
Norm of gradient: 0.20757479731910652
Iterations before filter: 30
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|10190.7992| 487.4735|9157.6109| 97.2364| 0.0000| 0.0000| 0.0000| 24.4880
Norm of gradient: 0.43336046078081397
Iterations before filter: 40
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|9575.0206| 543.6499|7723.6027| 105.1525| 0.0000| 0.0000| 0.0000| 26.2811
Norm of gradient: 0.18817372003299399
Iterations before filter: 50
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|8235.4327| 646.6531|7694.9368| 117.4818| 0.0000| 0.0000| 0.0000| 29.3711
Norm of gradient: 0.25028279630078826
Iterations before filter: 60
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|8043.5445| 676.6428|6947.9072| 121.7306| 0.0000| 0.0000| 0.0000| 30.3602
Norm of gradient: 0.08771931304200828
Iterations before filter: 70
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|7279.2288| 750.4795|6909.7738| 130.9263| 0.0000| 0.0000| 0.0000| 32.7090
Norm of gradient: 0.08472728964816935
Iterations before filter: 80
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|7208.0899| 766.9357|6444.3950| 133.5794| 0.0000| 0.0000| 0.0000| 33.3331
Norm of gradient: 0.11231365381270392
Iterations before filter: 90
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|6896.6080| 794.1754|6397.3635| 137.3280| 0.0000| 0.0000| 0.0000| 34.3543
Norm of gradient: 0.1573375347164585
Iterations before filter: 100
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|6764.8440| 818.3751|6170.6504| 141.3587| 0.0000| 0.0000| 0.0000| 35.3335
Norm of gradient: 0.12371648539743063
Iterations before filter: 110
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|6617.6274| 826.8555|6031.3643| 142.5987| 0.0000| 0.0000| 0.0000| 35.7029
Norm of gradient: 0.03724475150710924
Iterations before filter: 120
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|6454.5022| 852.4353|5872.7575| 147.1010| 0.0000| 0.0000| 0.0000| 36.8066
Norm of gradient: 0.06588787031732482
Iterations before filter: 130
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|6325.0864| 860.8269|5754.1528| 148.5697| 0.0000| 0.0000| 0.0000| 37.2212
Norm of gradient: 0.07474420371084677
Iterations before filter: 140
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|6009.6687| 912.4590|5574.8414| 158.1301| 0.0000| 0.0000| 0.0000| 39.4422
Norm of gradient: 0.16677426737873463
Iterations before filter: 150
ERROR:root:Internal Python error in the inspect module.
Below is the traceback from this internal error.
Traceback (most recent call last):
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/interactiveshell.py", line 3331, in run_code
exec(code_obj, self.user_global_ns, self.user_ns)
File "<ipython-input-15-4b869932aad0>", line 2, in <module>
dual_doppler_analysis(time, smooth_param=0.5, use_sounding=True, second_param=0.01)
File "<ipython-input-11-6cd94a096c02>", line 58, in dual_doppler_analysis
mask_outside_opt=True)
File "/Users/mgrover/git_repos/PyDDA/pydda/retrieval/wind_retrieve.py", line 518, in get_dd_wind_field
fprime=grad_J, disp=0, iprint=-1)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/lbfgsb.py", line 198, in fmin_l_bfgs_b
**opts)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/lbfgsb.py", line 360, in _minimize_lbfgsb
f, g = func_and_grad(x)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py", line 201, in fun_and_grad
self._update_grad()
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py", line 171, in _update_grad
self._update_grad_impl()
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py", line 85, in update_grad
self.g = grad_wrapped(self.x)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py", line 82, in grad_wrapped
return np.atleast_1d(grad(x, *args))
File "/Users/mgrover/git_repos/PyDDA/pydda/cost_functions/cost_functions.py", line 121, in grad_J
parameters.rmsVr, coeff=parameters.Co, upper_bc=parameters.upper_bc)
File "/Users/mgrover/git_repos/PyDDA/pydda/cost_functions/cost_functions.py", line 279, in calculate_grad_radial_vel
np.sin(azs[i]) * weights[i]) * lambda_o
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/numpy/ma/core.py", line 3031, in __array_wrap__
def __array_wrap__(self, obj, context=None):
KeyboardInterrupt
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/interactiveshell.py", line 2044, in showtraceback
stb = value._render_traceback_()
AttributeError: 'KeyboardInterrupt' object has no attribute '_render_traceback_'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/ultratb.py", line 1148, in get_records
return _fixed_getinnerframes(etb, number_of_lines_of_context, tb_offset)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/ultratb.py", line 316, in wrapped
return f(*args, **kwargs)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/ultratb.py", line 350, in _fixed_getinnerframes
records = fix_frame_records_filenames(inspect.getinnerframes(etb, context))
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/inspect.py", line 1502, in getinnerframes
frameinfo = (tb.tb_frame,) + getframeinfo(tb, context)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/inspect.py", line 1460, in getframeinfo
filename = getsourcefile(frame) or getfile(frame)
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/inspect.py", line 696, in getsourcefile
if getattr(getmodule(object, filename), '__loader__', None) is not None:
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/inspect.py", line 742, in getmodule
os.path.realpath(f)] = module.__name__
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/posixpath.py", line 395, in realpath
path, ok = _joinrealpath(filename[:0], filename, {})
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/posixpath.py", line 429, in _joinrealpath
if not islink(newpath):
File "/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/posixpath.py", line 171, in islink
st = os.lstat(path)
KeyboardInterrupt
---------------------------------------------------------------------------
def dual_doppler_analysis(time, smooth_param=0.02, use_sounding=True, second_param=1, case='Nov10', radar1='DOW6', radar2='DOW7'):
"""
Runs dual doppler analysis using the specified times
"""
# Read in the files
dow6_files = glob.glob(f'output/{case}/{radar1}/v{time}/*')
dow7_files = glob.glob(f'output/{case}/{radar2}/v{time}/*')
# Read in the sounding
#sounding = pyart.io.read_arm_sonde('soundings/corsondewnpnS1.b1.20181110.180000.custom.cdf')
# Grid the radar data using the function above
dow7_grid, dow6_grid = grid_radars(dow7_files[0], dow6_files[0])
# Make sure only one value is used for each radar lat, lon, and altitude
dow6_grid.radar_latitude['data'] = dow6_grid.radar_latitude['data'][0:1]
dow6_grid.radar_longitude['data'] = dow6_grid.radar_longitude['data'][0:1]
dow6_grid.radar_altitude['data'] = dow6_grid.radar_altitude['data'][0:1]
dow7_grid.origin_altitude['data'] = dow7_grid.origin_altitude['data'][0:1]
dow7_grid.origin_latitude['data'] = dow7_grid.origin_latitude['data'][0:1]
dow7_grid.origin_longitude['data'] = dow7_grid.origin_longitude['data'][0:1]
# Use ERA data to constrain the velocity field intialization
dow6_grid = pydda.constraints.make_constraint_from_era_interim(dow6_grid, vel_field='VM')
if use_sounding:
# Load sounding data and insert as an intialization
u_init, v_init, w_init = pydda.initialization.make_wind_field_from_profile(dow6_grid, sounding[1], vel_field='VM')
else:
# Otherwise use constant wind field
u_init, v_init, w_init = pydda.initialization.make_constant_wind_field(dow6_grid, vel_field='VM')
# Run the dual-doppler retrieval
new_grids = pydda.retrieval.get_dd_wind_field([dow7_grid, dow6_grid],
u_init, v_init, w_init, vel_name='VM', refl_field='ZM',
Co=1., Cm=1000., Cx=second_param, Cy=second_param, upper_bc=True,
Cv=.001, Ut = 7.4, Vt = -13.34,
mask_outside_opt=True)
# Write the first grid to netcdf containing the dual doppler analysis
new_grids[1].to_xarray().reset_coords().to_netcdf(f'dual_output/{case}/vort_constraint_{time}_smooth_param_{smooth_param}.nc')
###################################
# Plot 6 km Vertical Velocity (w)
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='w', level=12,
show_lobes=True, bg_grid_no=1, vmin=-30, vmax=30,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=20, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='seismic')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'{case} 2018 {time} UTC 6 km /n Smooth Param: {smooth_param}', fontsize=16)
plt.savefig(f'plots/{case}/vort_constraint_vert_velocity_{time}_utc_6km_smooth_param_{smooth_param}.png', dpi=200)
# Close out the figure
plt.close()
##############################
# Plot 3 km Vertical Velocity
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='w', level=6,
show_lobes=True, bg_grid_no=1, vmin=-17, vmax=17,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='seismic')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'{case} 2018 {time} UTC 3 km /n Smooth Param: {smooth_param}', fontsize=16)
plt.savefig(f'plots/{case}/vort_constraint_vert_velocity_{time}_utc_3km_smooth_param_{smooth_param}.png', dpi=200)
plt.close()
#############################
# Plot 3 km Reflectivity
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='ZM', level=6,
show_lobes=True, bg_grid_no=1, vmin=0, vmax=70,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='pyart_HomeyerRainbow')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'{case} 2018 {time} UTC 3 km /n Smooth Param: {smooth_param}', fontsize=16)
plt.savefig(f'plots/{case}/vort_constraint_reflectivity_{time}_utc_3km_smooth_param_{smooth_param}.png', dpi=200)
plt.close()
#############################
# Plot 3 km Radial Velocity
fig = plt.figure(figsize=(10,7))
ax = pydda.vis.plot_horiz_xsection_quiver_map(new_grids, background_field='VM', level=10,
show_lobes=True, bg_grid_no=1, vmin=-30, vmax=30,
colorbar_contour_flag=True, contour_alpha=0.7,
quiverkey_len=15, quiver_spacing_x_km=2, quiver_spacing_y_km=2,
quiver_width=0.003, cmap='seismic')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.title(f'10 November 2018 {time} UTC 3 km /n Smooth Param: {smooth_param}', fontsize=16)
plt.savefig(f'plots/vort_constraint_vel_{time}_utc_3km_smooth_param_{smooth_param}.png', dpi=200)
for time in times:
dual_doppler_analysis(time, smooth_param=0.5, use_sounding=False, second_param=0)
Download ERA Interim data...
2020-08-31 13:28:43 ECMWF API python library 1.5.4
2020-08-31 13:28:43 ECMWF API at https://api.ecmwf.int/v1
2020-08-31 13:28:44 Welcome Maxwell Grover
2020-08-31 13:28:44 In case of problems, please check https://confluence.ecmwf.int/display/WEBAPI/Web+API+FAQ or contact servicedesk@ecmwf.int
2020-08-31 13:28:45 Request submitted
2020-08-31 13:28:45 Request id: 5f4d415d97446011ecb05c6c
2020-08-31 13:28:45 Request is submitted
2020-08-31 13:28:46 Request is active
Calling 'nice mars /tmp/20200831-1820/2d/tmp-_marscZUlTA.req'
mars - WARN -
mars - WARN - From 29 January 2019 10AM (UTC) MARS uses the interpolation
mars - WARN - provided by the MIR library. For more details, see
mars - WARN - https://confluence.ecmwf.int/display/UDOC/MARS+interpolation+with+MIR
mars - WARN -
MIR environment variables:
MIR_CACHE_PATH=/data/ec_coeff
mars - INFO - 20200831.182846 - Welcome to MARS
mars - INFO - 20200831.182846 - MARS Client bundle version: 6.28.6.1
mars - INFO - 20200831.182846 - MARS Client package version: 6.28.6
mars - INFO - 20200831.182846 - MARS Client build stamp: 20200717102127
mars - INFO - 20200831.182846 - MIR version: 1.4.7
mars - INFO - 20200831.182846 - Using ecCodes version 2.18.0
mars - INFO - 20200831.182846 - Using odb_api version: 0.15.11 (file format version: 0.5)
mars - INFO - 20200831.182846 - Using FDB5 version: 5.6.1
mars - INFO - 20200831.182846 - Maximum retrieval size is 50.00 G
retrieve,stream=oper,levelist=1/2/3/5/7/10/20/30/50/70/100/125/150/175/200/225/250/300/350/400/450/500/550/600/650/700/750/775/800/825/850/875/900/925/950/975/1000,area=-31.8/-64.5/-32.4/-63.9,levtype=pl,param=131.128/132.128/135.128/129.128,padding=0,step=0,grid=0.75/0.75,expver=0001,time=18,date=2018-11-10,class=eimars - WARN - 20200831.182846 - For full resolution grid, it is recommended to use RESOL=AV to prevent any truncation before transformation
mars - INFO - 20200831.182846 - Automatic split by date is on
mars - INFO - 20200831.182846 - Processing request 1
RETRIEVE,
CLASS = EI,
TYPE = AN,
STREAM = OPER,
EXPVER = 0001,
REPRES = SH,
LEVTYPE = PL,
LEVELIST = 1/2/3/5/7/10/20/30/50/70/100/125/150/175/200/225/250/300/350/400/450/500/550/600/650/700/750/775/800/825/850/875/900/925/950/975/1000,
PARAM = 131.128/132.128/135.128/129.128,
TIME = 1800,
STEP = 0,
DOMAIN = G,
RESOL = AUTO,
AREA = -31.8/-64.5/-32.4/-63.9,
GRID = 0.75/0.75,
PADDING = 0,
DATE = 20181110
mars - INFO - 20200831.182846 - Web API request id: 5f4d415d97446011ecb05c6c
mars - INFO - 20200831.182846 - Requesting 148 fields
mars - INFO - 20200831.182846 - Calling mars on 'marser', local port is 60990
mars - INFO - 20200831.182846 - Server task is 365 [marser]
mars - INFO - 20200831.182846 - Request cost: 148 fields, 18.7188 Mbytes online, nodes: mvr06 [marser]
mars - INFO - 20200831.182846 - Wind conversion requested by server
mars - INFO - 20200831.182846 - Transfering 19628056 bytes
mars - INFO - 20200831.182847 - ShToGridded: loading Legendre coefficients '/data/ec_coeff/mir/legendre/4/local-T239-GaussianN120-OPT4189816c2e.leg'
mars - INFO - 20200831.182847 - Deriving U and V from vorticity and divergence
mars - INFO - 20200831.182849 - 148 fields retrieved from 'marser'
mars - INFO - 20200831.182849 - 148 fields have been interpolated
mars - INFO - 20200831.182849 - Request time: wall: 3 sec cpu: 2 sec
mars - INFO - 20200831.182849 - Read from network: 18.72 Mbyte(s) in < 1 sec [37.06 Mbyte/sec]
mars - INFO - 20200831.182849 - Visiting marser: wall: 3 sec
mars - INFO - 20200831.182849 - Post-processing: wall: 2 sec cpu: 2 sec
mars - INFO - 20200831.182849 - Writing to target file: 15.90 Kbyte(s) in < 1 sec [1014.00 Kbyte/sec]
mars - INFO - 20200831.182849 - Memory used: 44.61 Mbyte(s)
mars - INFO - 20200831.182849 - No errors reported
Process '['nice', 'mars', '/tmp/20200831-1820/2d/tmp-_marscZUlTA.req']' finished
Calling 'nice grib_to_netcdf /data/scratch/20200831-1820/9f/_mars-webmars-public-svc-blue-001-6fe5cac1a363ec1525f54343b6cc9fd8-Klc4hj.grib -o /data/scratch/20200831-1820/43/_grib2netcdf-webmars-public-svc-blue-000-6fe5cac1a363ec1525f54343b6cc9fd8-nXp11D.nc -utime'
grib_to_netcdf: Version 2.18.0
grib_to_netcdf: Processing input file '/data/scratch/20200831-1820/9f/_mars-webmars-public-svc-blue-001-6fe5cac1a363ec1525f54343b6cc9fd8-Klc4hj.grib'.
grib_to_netcdf: Found 148 GRIB fields in 1 file.
grib_to_netcdf: Ignoring key(s): method, type, stream, refdate, hdate
grib_to_netcdf: Creating netCDF file '/data/scratch/20200831-1820/43/_grib2netcdf-webmars-public-svc-blue-000-6fe5cac1a363ec1525f54343b6cc9fd8-nXp11D.nc'
grib_to_netcdf: NetCDF library version: 4.3.3.1 of Dec 10 2015 16:44:18 $
grib_to_netcdf: Creating large (64 bit) file format.
grib_to_netcdf: Defining variable 'w'.
grib_to_netcdf: Defining variable 'z'.
grib_to_netcdf: Defining variable 'u'.
grib_to_netcdf: Defining variable 'v'.
grib_to_netcdf: Done.
Process '['nice', 'grib_to_netcdf', '/data/scratch/20200831-1820/9f/_mars-webmars-public-svc-blue-001-6fe5cac1a363ec1525f54343b6cc9fd8-Klc4hj.grib', '-o', '/data/scratch/20200831-1820/43/_grib2netcdf-webmars-public-svc-blue-000-6fe5cac1a363ec1525f54343b6cc9fd8-nXp11D.nc', '-utime']' finished
2020-08-31 13:28:52 Request is complete
2020-08-31 13:28:52 Transfering 2.53125 Kbytes into /var/folders/dd/zgpcpccj46q2tfrvpwpp3r5w0000gn/T/tmp8n43cbma
2020-08-31 13:28:52 From https://stream.ecmwf.int/data/webmars-public-svc-blue-000/data/scratch/20200831-1820/43/_grib2netcdf-webmars-public-svc-blue-000-6fe5cac1a363ec1525f54343b6cc9fd8-nXp11D.nc
2020-08-31 13:28:52 Transfer rate 4.87089 Kbytes/s
Calculating weights for radars 0 and 1
Calculating weights for models...
Starting solver
rmsVR = 21.272561224299345
Total points: 165400
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:408: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:409: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:419: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:420: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] <= math.radians(max_bca))] = 1
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca))] = 1
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 146.6696| 637.9176| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 85.1680
Norm of gradient: 0.07935328058422833
Iterations before filter: 10
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 87.2911| 529.1742| 0.0000| 0.0000| 0.0001| 0.0000| 0.0000| 93.7495
Norm of gradient: 0.08120502082651836
Iterations before filter: 20
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 53.6721| 513.9433| 0.0000| 0.0000| 0.0001| 0.0000| 0.0000| 97.2404
Norm of gradient: 0.04814619965261029
Iterations before filter: 30
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 53.2962| 514.1742| 0.0000| 0.0000| 0.0001| 0.0000| 0.0000| 97.2578
Norm of gradient: 0.04830782412857658
Iterations before filter: 40
Applying low pass filter to wind field...
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|8293.2121| 446.0019| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 46.3354
Norm of gradient: 0.1715791648029771
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
|8219.3997| 445.2171| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 46.3573
Norm of gradient: 0.17022850139143314
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 393.2368| 813.4342| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 52.1118
Norm of gradient: 0.05726674095032224
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 216.0191| 747.0091| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 52.5758
Norm of gradient: 0.06698429741755814
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 173.3670| 585.9617| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 51.9593
Norm of gradient: 0.044854444375534354
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 137.8444| 484.6339| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 56.7010
Norm of gradient: 0.03288516373681457
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 112.1064| 468.6932| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 62.1934
Norm of gradient: 0.03559839686258209
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 124.2240| 536.3347| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 76.2040
Norm of gradient: 0.07601957900013732
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 99.4757| 460.0264| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 65.6137
Norm of gradient: 0.03823731589869442
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 114.1317| 561.6280| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 84.0764
Norm of gradient: 0.06729642310466184
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 86.7073| 455.7837| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 69.5424
Norm of gradient: 0.03980122641343633
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 161.8409| 624.3505| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 96.9268
Norm of gradient: 0.07138129235922891
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 77.6954| 449.9467| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 74.9320
Norm of gradient: 0.04077582781855498
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 144.4209| 605.2767| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 100.0000
Norm of gradient: 0.05377274253622062
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 72.5019| 449.8673| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 79.4383
Norm of gradient: 0.04078717198483486
Iterations after filter: 1
Iterations after filter: 2
Done! Time = 210.7
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: divide by zero encountered in true_divide
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/cartopy/mpl/geoaxes.py:1478: UserWarning: The following kwargs were not used by contour: 'color'
result = matplotlib.axes.Axes.contour(self, *args, **kwargs)
Download ERA Interim data...
2020-08-31 13:33:58 ECMWF API python library 1.5.4
2020-08-31 13:33:58 ECMWF API at https://api.ecmwf.int/v1
2020-08-31 13:33:58 Welcome Maxwell Grover
2020-08-31 13:33:59 In case of problems, please check https://confluence.ecmwf.int/display/WEBAPI/Web+API+FAQ or contact servicedesk@ecmwf.int
2020-08-31 13:33:59 Request submitted
2020-08-31 13:33:59 Request id: 5f4d4297cb8af4f322b05c74
2020-08-31 13:33:59 Request is submitted
2020-08-31 13:34:01 Request is active
Calling 'nice mars /tmp/20200831-1830/ef/tmp-_marsiWVvn_.req'
mars - WARN -
mars - WARN - From 29 January 2019 10AM (UTC) MARS uses the interpolation
mars - WARN - provided by the MIR library. For more details, see
mars - WARN - https://confluence.ecmwf.int/display/UDOC/MARS+interpolation+with+MIR
mars - WARN -
MIR environment variables:
MIR_CACHE_PATH=/data/ec_coeff
mars - INFO - 20200831.183400 - Welcome to MARS
mars - INFO - 20200831.183400 - MARS Client bundle version: 6.28.6.1
mars - INFO - 20200831.183400 - MARS Client package version: 6.28.6
mars - INFO - 20200831.183400 - MARS Client build stamp: 20200717102127
mars - INFO - 20200831.183400 - MIR version: 1.4.7
mars - INFO - 20200831.183400 - Using ecCodes version 2.18.0
mars - INFO - 20200831.183400 - Using odb_api version: 0.15.11 (file format version: 0.5)
mars - INFO - 20200831.183400 - Using FDB5 version: 5.6.1
mars - INFO - 20200831.183400 - Maximum retrieval size is 50.00 G
retrieve,stream=oper,levelist=1/2/3/5/7/10/20/30/50/70/100/125/150/175/200/225/250/300/350/400/450/500/550/600/650/700/750/775/800/825/850/875/900/925/950/975/1000,area=-31.8/-64.5/-32.4/-63.9,levtype=pl,param=131.128/132.128/135.128/129.128,padding=0,step=0,grid=0.75/0.75,expver=0001,time=18,date=2018-11-10,class=eimars - WARN - 20200831.183400 - For full resolution grid, it is recommended to use RESOL=AV to prevent any truncation before transformation
mars - INFO - 20200831.183400 - Automatic split by date is on
mars - INFO - 20200831.183400 - Processing request 1
RETRIEVE,
CLASS = EI,
TYPE = AN,
STREAM = OPER,
EXPVER = 0001,
REPRES = SH,
LEVTYPE = PL,
LEVELIST = 1/2/3/5/7/10/20/30/50/70/100/125/150/175/200/225/250/300/350/400/450/500/550/600/650/700/750/775/800/825/850/875/900/925/950/975/1000,
PARAM = 131.128/132.128/135.128/129.128,
TIME = 1800,
STEP = 0,
DOMAIN = G,
RESOL = AUTO,
AREA = -31.8/-64.5/-32.4/-63.9,
GRID = 0.75/0.75,
PADDING = 0,
DATE = 20181110
mars - INFO - 20200831.183400 - Web API request id: 5f4d4297cb8af4f322b05c74
mars - INFO - 20200831.183400 - Requesting 148 fields
mars - INFO - 20200831.183400 - Calling mars on 'marser', local port is 39257
mars - INFO - 20200831.183400 - Server task is 965 [marser]
mars - INFO - 20200831.183400 - Request cost: 148 fields, 18.7188 Mbytes online, nodes: mvr06 [marser]
mars - INFO - 20200831.183400 - Wind conversion requested by server
mars - INFO - 20200831.183400 - Transfering 19628056 bytes
mars - INFO - 20200831.183400 - ShToGridded: loading Legendre coefficients '/data/ec_coeff/mir/legendre/4/local-T239-GaussianN120-OPT4189816c2e.leg'
mars - INFO - 20200831.183401 - Deriving U and V from vorticity and divergence
mars - INFO - 20200831.183403 - 148 fields retrieved from 'marser'
mars - INFO - 20200831.183403 - 148 fields have been interpolated
mars - INFO - 20200831.183403 - Request time: wall: 3 sec cpu: 2 sec
mars - INFO - 20200831.183403 - Read from network: 18.72 Mbyte(s) in < 1 sec [141.33 Mbyte/sec]
mars - INFO - 20200831.183403 - Visiting marser: wall: 3 sec
mars - INFO - 20200831.183403 - Post-processing: wall: 2 sec cpu: 2 sec
mars - INFO - 20200831.183403 - Writing to target file: 15.90 Kbyte(s) in < 1 sec [406.09 Kbyte/sec]
mars - INFO - 20200831.183403 - Memory used: 44.61 Mbyte(s)
mars - INFO - 20200831.183403 - No errors reported
Process '['nice', 'mars', '/tmp/20200831-1830/ef/tmp-_marsiWVvn_.req']' finished
Calling 'nice grib_to_netcdf /data/scratch/20200831-1830/07/_mars-webmars-public-svc-blue-004-6fe5cac1a363ec1525f54343b6cc9fd8-wh5f2A.grib -o /data/scratch/20200831-1830/d3/_grib2netcdf-webmars-public-svc-blue-006-6fe5cac1a363ec1525f54343b6cc9fd8-s8QgVj.nc -utime'
grib_to_netcdf: Version 2.18.0
grib_to_netcdf: Processing input file '/data/scratch/20200831-1830/07/_mars-webmars-public-svc-blue-004-6fe5cac1a363ec1525f54343b6cc9fd8-wh5f2A.grib'.
grib_to_netcdf: Found 148 GRIB fields in 1 file.
grib_to_netcdf: Ignoring key(s): method, type, stream, refdate, hdate
grib_to_netcdf: Creating netCDF file '/data/scratch/20200831-1830/d3/_grib2netcdf-webmars-public-svc-blue-006-6fe5cac1a363ec1525f54343b6cc9fd8-s8QgVj.nc'
grib_to_netcdf: NetCDF library version: 4.3.3.1 of Dec 10 2015 16:44:18 $
grib_to_netcdf: Creating large (64 bit) file format.
grib_to_netcdf: Defining variable 'w'.
grib_to_netcdf: Defining variable 'z'.
grib_to_netcdf: Defining variable 'u'.
grib_to_netcdf: Defining variable 'v'.
grib_to_netcdf: Done.
Process '['nice', 'grib_to_netcdf', '/data/scratch/20200831-1830/07/_mars-webmars-public-svc-blue-004-6fe5cac1a363ec1525f54343b6cc9fd8-wh5f2A.grib', '-o', '/data/scratch/20200831-1830/d3/_grib2netcdf-webmars-public-svc-blue-006-6fe5cac1a363ec1525f54343b6cc9fd8-s8QgVj.nc', '-utime']' finished
2020-08-31 13:34:07 Request is complete
2020-08-31 13:34:07 Transfering 2.53125 Kbytes into /var/folders/dd/zgpcpccj46q2tfrvpwpp3r5w0000gn/T/tmpq9ge7b7p
2020-08-31 13:34:07 From https://stream.ecmwf.int/data/webmars-public-svc-blue-006/data/scratch/20200831-1830/d3/_grib2netcdf-webmars-public-svc-blue-006-6fe5cac1a363ec1525f54343b6cc9fd8-s8QgVj.nc
2020-08-31 13:34:07 Transfer rate 4.92714 Kbytes/s
Calculating weights for radars 0 and 1
Calculating weights for models...
Starting solver
rmsVR = 20.837894214037224
Total points: 160055
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:652: RuntimeWarning: invalid value encountered in true_divide
theta_1 = np.arccos(x/a)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:653: RuntimeWarning: invalid value encountered in arccos
theta_2 = np.arccos((x-rad2[1])/b)
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:654: RuntimeWarning: invalid value encountered in true_divide
return np.arccos((a*a+b*b-c*c)/(2*a*b))
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:408: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:409: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:419: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] >= math.radians(min_bca),
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:420: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca)))] += 1
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in greater_equal
bca[i, j] <= math.radians(max_bca))] = 1
/Users/mgrover/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py:429: RuntimeWarning: invalid value encountered in less_equal
bca[i, j] <= math.radians(max_bca))] = 1
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 142.5886| 694.1900| 0.0000| 0.0000| 0.0000| 0.0000| 0.0000| 80.6109
Norm of gradient: 0.3180886525353942
Iterations before filter: 10
| Jvel | Jmass | Jsmooth | Jbg | Jvort | Jmodel | Jpoint | Max w
| 91.8737| 578.0423| 0.0000| 0.0000| 0.0001| 0.0000| 0.0000| 92.4386
Norm of gradient: 0.2913748260632707
Iterations before filter: 20
---------------------------------------------------------------------------
KeyboardInterrupt Traceback (most recent call last)
<ipython-input-35-bf3a023e5c83> in <module>
1 for time in times:
----> 2 dual_doppler_analysis(time, smooth_param=0.5, use_sounding=False, second_param=0)
<ipython-input-34-8566b7670636> in dual_doppler_analysis(time, smooth_param, use_sounding, second_param, case, radar1, radar2)
41 Co=1., Cm=1000., Cx=second_param, Cy=second_param, upper_bc=True,
42 Cv=.001, Ut = 7.4, Vt = -13.34,
---> 43 mask_outside_opt=True)
44
45 # Write the first grid to netcdf containing the dual doppler analysis
~/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/retrieval/wind_retrieve.py in get_dd_wind_field(Grids, u_init, v_init, w_init, points, vel_name, refl_field, u_back, v_back, z_back, frz, Co, Cm, Cx, Cy, Cz, Cb, Cv, Cmod, Cpoint, Ut, Vt, filt_iterations, mask_outside_opt, weights_obs, weights_model, weights_bg, max_iterations, mask_w_outside_opt, filter_window, filter_order, min_bca, max_bca, upper_bc, model_fields, output_cost_functions, roi)
516 winds = fmin_l_bfgs_b(J_function, winds, args=(parameters,),
517 maxiter=10, pgtol=1e-3, bounds=bounds,
--> 518 fprime=grad_J, disp=0, iprint=-1)
519 parameters.print_out = True
520 if output_cost_functions is True:
~/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/lbfgsb.py in fmin_l_bfgs_b(func, x0, fprime, args, approx_grad, bounds, m, factr, pgtol, epsilon, iprint, maxfun, maxiter, disp, callback, maxls)
196
197 res = _minimize_lbfgsb(fun, x0, args=args, jac=jac, bounds=bounds,
--> 198 **opts)
199 d = {'grad': res['jac'],
200 'task': res['message'],
~/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/lbfgsb.py in _minimize_lbfgsb(fun, x0, args, jac, bounds, disp, maxcor, ftol, gtol, eps, maxfun, maxiter, iprint, callback, maxls, finite_diff_rel_step, **unknown_options)
358 # until the completion of the current minimization iteration.
359 # Overwrite f and g:
--> 360 f, g = func_and_grad(x)
361 elif task_str.startswith(b'NEW_X'):
362 # new iteration
~/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py in fun_and_grad(self, x)
199 self._update_x_impl(x)
200 self._update_fun()
--> 201 self._update_grad()
202 return self.f, self.g
203
~/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py in _update_grad(self)
169 def _update_grad(self):
170 if not self.g_updated:
--> 171 self._update_grad_impl()
172 self.g_updated = True
173
~/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py in update_grad()
83
84 def update_grad():
---> 85 self.g = grad_wrapped(self.x)
86
87 elif grad in FD_METHODS:
~/miniconda3/envs/unidata/lib/python3.7/site-packages/scipy/optimize/_differentiable_functions.py in grad_wrapped(x)
80 def grad_wrapped(x):
81 self.ngev += 1
---> 82 return np.atleast_1d(grad(x, *args))
83
84 def update_grad():
~/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/cost_functions/cost_functions.py in grad_J(winds, parameters)
129 winds[0], winds[1], winds[2], parameters.z,
130 parameters.dx, parameters.dy, parameters.dz,
--> 131 coeff=parameters.Cm, upper_bc=parameters.upper_bc)
132
133 if(parameters.Cx > 0 or parameters.Cy > 0 or parameters.Cz > 0):
~/miniconda3/envs/unidata/lib/python3.7/site-packages/pydda/cost_functions/cost_functions.py in calculate_mass_continuity_gradient(u, v, w, z, dx, dy, dz, coeff, anel, upper_bc)
611 grad_u = -np.gradient(div2, dx, axis=2)*coeff
612 grad_v = -np.gradient(div2, dy, axis=1)*coeff
--> 613 grad_w = -np.gradient(div2, dz, axis=0)*coeff
614
615 # Impermeability condition
<__array_function__ internals> in gradient(*args, **kwargs)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/numpy/lib/function_base.py in gradient(f, *varargs, **kwargs)
1055
1056 if uniform_spacing:
-> 1057 out[tuple(slice1)] = (f[tuple(slice4)] - f[tuple(slice2)]) / (2. * ax_dx)
1058 else:
1059 dx1 = ax_dx[0:-1]
KeyboardInterrupt:
Error in callback <function flush_figures at 0x7f83dd9d9dd0> (for post_execute):
---------------------------------------------------------------------------
KeyboardInterrupt Traceback (most recent call last)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/ipykernel/pylab/backend_inline.py in flush_figures()
119 # ignore the tracking, just draw and close all figures
120 try:
--> 121 return show(True)
122 except Exception as e:
123 # safely show traceback if in IPython, else raise
~/miniconda3/envs/unidata/lib/python3.7/site-packages/ipykernel/pylab/backend_inline.py in show(close, block)
41 display(
42 figure_manager.canvas.figure,
---> 43 metadata=_fetch_figure_metadata(figure_manager.canvas.figure)
44 )
45 finally:
~/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/display.py in display(include, exclude, metadata, transient, display_id, *objs, **kwargs)
311 publish_display_data(data=obj, metadata=metadata, **kwargs)
312 else:
--> 313 format_dict, md_dict = format(obj, include=include, exclude=exclude)
314 if not format_dict:
315 # nothing to display (e.g. _ipython_display_ took over)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/formatters.py in format(self, obj, include, exclude)
178 md = None
179 try:
--> 180 data = formatter(obj)
181 except:
182 # FIXME: log the exception
<decorator-gen-9> in __call__(self, obj)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/formatters.py in catch_format_error(method, self, *args, **kwargs)
222 """show traceback on failed format call"""
223 try:
--> 224 r = method(self, *args, **kwargs)
225 except NotImplementedError:
226 # don't warn on NotImplementedErrors
~/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/formatters.py in __call__(self, obj)
339 pass
340 else:
--> 341 return printer(obj)
342 # Finally look for special method names
343 method = get_real_method(obj, self.print_method)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/pylabtools.py in <lambda>(fig)
246
247 if 'png' in formats:
--> 248 png_formatter.for_type(Figure, lambda fig: print_figure(fig, 'png', **kwargs))
249 if 'retina' in formats or 'png2x' in formats:
250 png_formatter.for_type(Figure, lambda fig: retina_figure(fig, **kwargs))
~/miniconda3/envs/unidata/lib/python3.7/site-packages/IPython/core/pylabtools.py in print_figure(fig, fmt, bbox_inches, **kwargs)
130 FigureCanvasBase(fig)
131
--> 132 fig.canvas.print_figure(bytes_io, **kw)
133 data = bytes_io.getvalue()
134 if fmt == 'svg':
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/backend_bases.py in print_figure(self, filename, dpi, facecolor, edgecolor, orientation, format, bbox_inches, **kwargs)
2101 bbox_artists = kwargs.pop("bbox_extra_artists", None)
2102 bbox_inches = self.figure.get_tightbbox(renderer,
-> 2103 bbox_extra_artists=bbox_artists)
2104 pad = kwargs.pop("pad_inches", None)
2105 if pad is None:
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/figure.py in get_tightbbox(self, renderer, bbox_extra_artists)
2393 try:
2394 bbox = ax.get_tightbbox(renderer,
-> 2395 bbox_extra_artists=bbox_extra_artists)
2396 except TypeError:
2397 bbox = ax.get_tightbbox(renderer)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/axes/_base.py in get_tightbbox(self, renderer, call_axes_locator, bbox_extra_artists)
4325 bb.append(bb_xaxis)
4326
-> 4327 bb_yaxis = self.yaxis.get_tightbbox(renderer)
4328 if bb_yaxis:
4329 bb.append(bb_yaxis)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/axis.py in get_tightbbox(self, renderer)
1184 return
1185
-> 1186 ticks_to_draw = self._update_ticks()
1187
1188 self._update_label_position(renderer)
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/axis.py in _update_ticks(self)
1101 the axes. Return the list of ticks that will be drawn.
1102 """
-> 1103 major_locs = self.get_majorticklocs()
1104 major_labels = self.major.formatter.format_ticks(major_locs)
1105 major_ticks = self.get_major_ticks(len(major_locs))
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/axis.py in get_majorticklocs(self)
1346 def get_majorticklocs(self):
1347 """Get the array of major tick locations in data coordinates."""
-> 1348 return self.major.locator()
1349
1350 def get_minorticklocs(self):
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/ticker.py in __call__(self)
2201 def __call__(self):
2202 vmin, vmax = self.axis.get_view_interval()
-> 2203 return self.tick_values(vmin, vmax)
2204
2205 def tick_values(self, vmin, vmax):
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/colorbar.py in tick_values(self, vmin, vmax)
245 vmin = max(vmin, self._colorbar.norm.vmin)
246 vmax = min(vmax, self._colorbar.norm.vmax)
--> 247 ticks = super().tick_values(vmin, vmax)
248 rtol = (vmax - vmin) * 1e-10
249 return ticks[(ticks >= vmin - rtol) & (ticks <= vmax + rtol)]
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/ticker.py in tick_values(self, vmin, vmax)
2209 vmin, vmax = mtransforms.nonsingular(
2210 vmin, vmax, expander=1e-13, tiny=1e-14)
-> 2211 locs = self._raw_ticks(vmin, vmax)
2212
2213 prune = self._prune
~/miniconda3/envs/unidata/lib/python3.7/site-packages/matplotlib/ticker.py in _raw_ticks(self, vmin, vmax)
2194 ticks = np.arange(low, high + 1) * step + best_vmin
2195 # Count only the ticks that will be displayed.
-> 2196 nticks = ((ticks <= _vmax) & (ticks >= _vmin)).sum()
2197 if nticks >= self._min_n_ticks:
2198 break
KeyboardInterrupt:
time = '2012'
dow6_files = glob.glob(f'output/DOW6/v{time}/*')
dow7_files = glob.glob(f'output/DOW7/v{time}/*')
smoothing_vals = np.arange(0, .11, .01)
for val in smoothing_vals:
dual_doppler_analysis('2014', val, use_sounding=True)
12 November Case¶
import glob
dow6_files = sorted(glob.glob('output/Nov12/DOW6/*'))
dow7_files = sorted(glob.glob('output/Nov12/DOW7/*'))
dow8_files = sorted(glob.glob('output/Nov12/DOW8/*'))
dow6_files
['output/Nov12/DOW6/cfrad.20181112_020007.258_to_20181112_020420.238_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_020431.790_to_20181112_020844.728_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_021007.906_to_20181112_021420.886_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_021432.438_to_20181112_021845.378_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_022007.270_to_20181112_022420.250_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_022431.802_to_20181112_022844.740_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_023007.920_to_20181112_023420.900_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_023432.450_to_20181112_023845.390_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_024007.296_to_20181112_024420.276_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_024431.826_to_20181112_024844.768_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_025007.946_to_20181112_025420.926_DOW6high_SUR.nc',
'output/Nov12/DOW6/cfrad.20181112_025432.478_to_20181112_025845.418_DOW6high_SUR.nc']
for i in range(len(dow6_files)):
# Cleanup the radar data
dow6_radar = cleanup_radar(dow6_files[i])
dow7_radar = cleanup_radar(dow7_files[i])
#dow8_radar = cleanup_radar(dow8_files[i])
# Use DOW7 as the central lat and lon for regridding
grid_lat = dow6_radar.latitude['data'][0]
grid_lon = dow6_radar.longitude['data'][0]
# Regrid the radars
#gridded_radars = grid_radars([dow6_radar, dow7_radar, dow8_radar], grid_lat=grid_lat, grid_lon=grid_lon, vert_extent=14500,
# horiz_extent=50000)
gridded_radars = grid_radars([dow6_radar, dow7_radar], grid_lat=grid_lat, grid_lon=grid_lon, vert_extent=14500,
horiz_extent=50000)
# Run the dual doppler analysis
run_dda(gridded_radars, case='Nov12')
grid_lat = dow7_radar.latitude['data'][0]
grid_lon = dow7_radar.longitude['data'][0]
gridded_radars = grid_radars([dow6_radar, dow7_radar, dow8_radar], grid_lat=grid_lat, grid_lon=grid_lon, vert_extent=14500,
horiz_extent=50000)
run_dda(gridded_radars, case='Nov12')
df = pd.read_csv('../ot_code/relampago_ots/20181112.02_1min.csv', index_col='time', parse_dates=True)
df.head(10)
4 December Case¶
import glob
dow6_files = glob.glob('output/Dec4/DOW6/*')
dow7_files = glob.glob('output/Dec4/DOW7/*')
dow8_files = glob.glob('output/Dec4/DOW8/*')
dow6_radar = cleanup_radar(dow6_files[0])
dow7_radar = cleanup_radar(dow7_files[0])
dow8_radar = cleanup_radar(dow8_files[0])
grid_lat = dow7_radar.latitude['data'][0]
grid_lon = dow7_radar.longitude['data'][0]
gridded_radars = grid_radars([dow6_radar, dow7_radar, dow8_radar], grid_lat=grid_lat, grid_lon=grid_lon,
horiz_extent=50000)
run_dda(gridded_radars, case='Dec4')